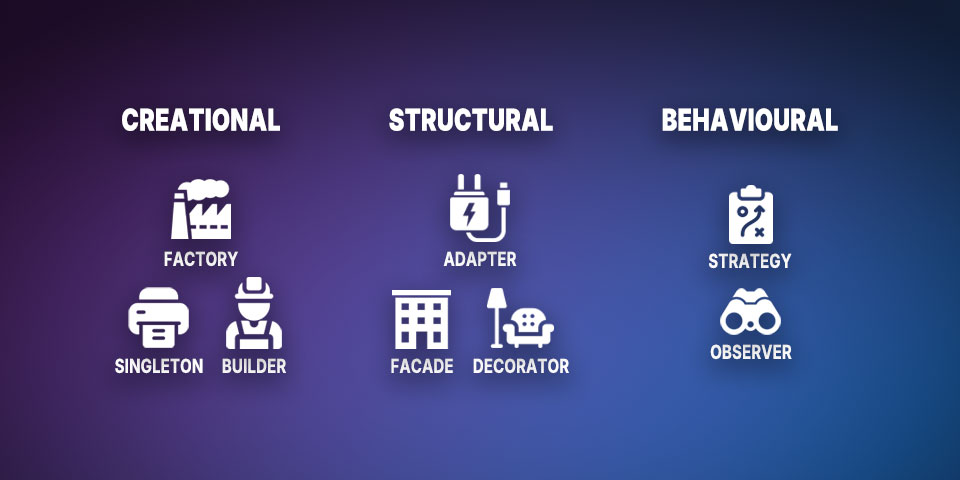
Software Design Patterns
In software engineering, design patterns are tried-and-true solutions to common design challenges. They aren’t code, but rather conceptual blueprints—templates you can adapt to solve problems consistently across projects.
By offering structured approaches to recurring design issues, design patterns help engineers build software that’s more scalable, maintainable, and collaborative.
What Makes Design Patterns Useful?
Reusability
Design patterns provide general solutions that can be applied across different problems and contexts, helping teams avoid reinventing the wheel.
Adaptability
These templates are flexible. You can tweak them to meet your project’s specific needs without starting from scratch.
Communication
Patterns create a shared vocabulary for discussing architecture and design, making it easier for teams to stay aligned.
Maintainability
Code built around patterns is typically more modular and easier to read, refactor, or extend.
Not Code
It’s important to remember: patterns are not finished code. They’re proven solutions to recurring design issues, offered in a form that’s abstract and broadly applicable.
OOP Alignment
Most patterns come from an object-oriented programming (OOP) context, solving problems related to class structure and object behavior.
Common Design Patterns
Here are a few foundational patterns you’ll encounter frequently:
-
Singleton
Ensures that a class has only one instance and provides a global access point to it. -
Factory Method
Defines an interface for object creation, letting subclasses decide which class to instantiate. -
Observer
Sets up a one-to-many relationship so when one object changes, all its dependents are automatically updated. -
Strategy
Encapsulates different algorithms or behaviors, allowing them to be swapped in and out at runtime. -
Decorator
Adds responsibilities to objects dynamically without modifying their code.
Why Use Design Patterns?
-
Improved Code Quality
Patterns encourage good practices like separation of concerns and modularity. -
Faster Development
You save time by relying on proven approaches rather than inventing solutions from scratch. -
Better Collaboration
Having a common design language makes team discussions more productive and less ambiguous. -
Reusable Solutions
Patterns are inherently reusable—across different teams, projects, and problem domains. -
Simplified Design
They break down complex problems into manageable components, leading to clearer and more maintainable architecture.
Design patterns won’t solve every issue, but when used appropriately, they bring clarity, consistency, and confidence to your software design process.